1The Reactive Extensions for C++ (__RxCpp__) is a library of algorithms for values-distributed-in-time. The [__Range-v3__](https://github.com/ericniebler/range-v3) library does the same for values-distributed-in-space. 2 3Platform | Status | 4----------- | :------------ | 5Windows | [](https://ci.appveyor.com/project/kirkshoop/rxcpp-446) 6Linux & OSX | [](https://travis-ci.org/ReactiveX/RxCpp) 7 8Source | Badges | 9------------- | :--------------- | 10Github | [](https://github.com/ReactiveX/RxCpp) <br/> [](https://github.com/ReactiveX/RxCpp/releases) <br/> [](https://github.com/ReactiveX/RxCpp) 11Gitter.im | [](https://gitter.im/ReactiveX/RxCpp?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge) 12Packages | [](http://www.nuget.org/packages/RxCpp/) [](https://github.com/Microsoft/vcpkg/tree/master/ports/rxcpp) 13Documentation | [](http://reactivex.github.io/RxCpp) <br/> [](http://reactivex.io/intro.html) [](http://rxmarbles.com/) 14 15# Usage 16 17__RxCpp__ is a header-only C++ library that only depends on the standard library. The CMake build generates documentation and unit tests. The unit tests depend on a git submodule for the [Catch](https://github.com/philsquared/Catch) library. 18 19# Example 20Add `Rx/v2/src` to the include paths 21 22[](http://kirkshoop.github.io/async/rxcpp/c++/2015/07/07/rxcpp_-_parsing_bytes_to_lines_of_text.html) 23 24```cpp 25#include "rxcpp/rx.hpp" 26namespace Rx { 27using namespace rxcpp; 28using namespace rxcpp::sources; 29using namespace rxcpp::operators; 30using namespace rxcpp::util; 31} 32using namespace Rx; 33 34#include <regex> 35#include <random> 36using namespace std; 37using namespace std::chrono; 38 39int main() 40{ 41 random_device rd; // non-deterministic generator 42 mt19937 gen(rd()); 43 uniform_int_distribution<> dist(4, 18); 44 45 // for testing purposes, produce byte stream that from lines of text 46 auto bytes = range(0, 10) | 47 flat_map([&](int i){ 48 auto body = from((uint8_t)('A' + i)) | 49 repeat(dist(gen)) | 50 as_dynamic(); 51 auto delim = from((uint8_t)'\r'); 52 return from(body, delim) | concat(); 53 }) | 54 window(17) | 55 flat_map([](observable<uint8_t> w){ 56 return w | 57 reduce( 58 vector<uint8_t>(), 59 [](vector<uint8_t> v, uint8_t b){ 60 v.push_back(b); 61 return v; 62 }) | 63 as_dynamic(); 64 }) | 65 tap([](vector<uint8_t>& v){ 66 // print input packet of bytes 67 copy(v.begin(), v.end(), ostream_iterator<long>(cout, " ")); 68 cout << endl; 69 }); 70 71 // 72 // recover lines of text from byte stream 73 // 74 75 auto removespaces = [](string s){ 76 s.erase(remove_if(s.begin(), s.end(), ::isspace), s.end()); 77 return s; 78 }; 79 80 // create strings split on \r 81 auto strings = bytes | 82 concat_map([](vector<uint8_t> v){ 83 string s(v.begin(), v.end()); 84 regex delim(R"/(\r)/"); 85 cregex_token_iterator cursor(&s[0], &s[0] + s.size(), delim, {-1, 0}); 86 cregex_token_iterator end; 87 vector<string> splits(cursor, end); 88 return iterate(move(splits)); 89 }) | 90 filter([](const string& s){ 91 return !s.empty(); 92 }) | 93 publish() | 94 ref_count(); 95 96 // filter to last string in each line 97 auto closes = strings | 98 filter( 99 [](const string& s){ 100 return s.back() == '\r'; 101 }) | 102 Rx::map([](const string&){return 0;}); 103 104 // group strings by line 105 auto linewindows = strings | 106 window_toggle(closes | start_with(0), [=](int){return closes;}); 107 108 // reduce the strings for a line into one string 109 auto lines = linewindows | 110 flat_map([&](observable<string> w) { 111 return w | start_with<string>("") | sum() | Rx::map(removespaces); 112 }); 113 114 // print result 115 lines | 116 subscribe<string>(println(cout)); 117 118 return 0; 119} 120``` 121 122# Reactive Extensions 123 124>The ReactiveX Observable model allows you to treat streams of asynchronous events with the same sort of simple, composable operations that you use for collections of data items like arrays. It frees you from tangled webs of callbacks, and thereby makes your code more readable and less prone to bugs. 125 126Credit [ReactiveX.io](http://reactivex.io/intro.html) 127 128### Other language implementations 129 130* Java: [RxJava](https://github.com/ReactiveX/RxJava) 131* JavaScript: [rxjs](https://github.com/ReactiveX/rxjs) 132* C#: [Rx.NET](https://github.com/Reactive-Extensions/Rx.NET) 133* [More..](http://reactivex.io/languages.html) 134 135### Resources 136 137* [Intro](http://reactivex.io/intro.html) 138* [Tutorials](http://reactivex.io/tutorials.html) 139* [Marble Diagrams](http://rxmarbles.com/) 140* [twitter stream analysis app](https://github.com/kirkshoop/twitter) 141 * [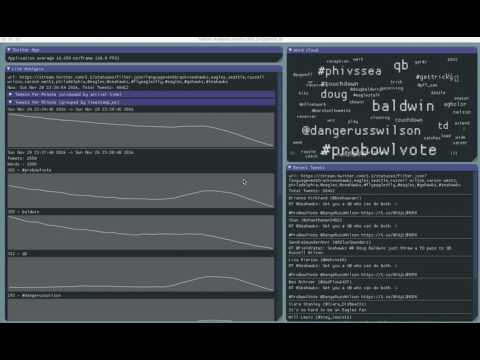](https://www.youtube.com/watch?v=QkvCzShHyVU) 142* _Algorithm Design For Values Distributed In Time_ 143 * [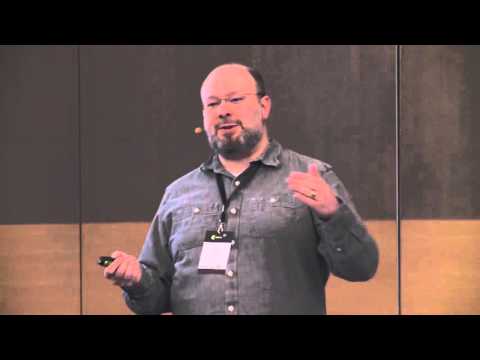](https://www.youtube.com/watch?v=Re6DS5Ff0uE) 144 * [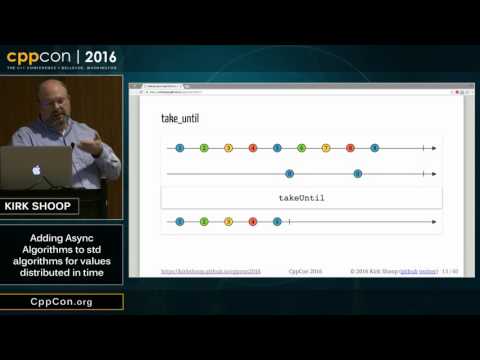](https://www.youtube.com/watch?v=FcQURwM806o) 145 146# Cloning RxCpp 147 148RxCpp uses a git submodule (in `ext/catch`) for the excellent [Catch](https://github.com/philsquared/Catch) library. The easiest way to ensure that the submodules are included in the clone is to add `--recursive` in the clone command. 149 150```shell 151git clone --recursive https://github.com/ReactiveX/RxCpp.git 152cd RxCpp 153``` 154 155# Building RxCpp Unit Tests 156 157* RxCpp is regularly tested on OSX and Windows. 158* RxCpp is regularly built with Clang, Gcc and VC 159* RxCpp depends on the latest compiler releases. 160 161RxCpp uses CMake to create build files for several platforms and IDE's 162 163### ide builds 164 165#### XCode 166```shell 167mkdir projects/build 168cd projects/build 169cmake -G"Xcode" ../CMake -B. 170``` 171 172#### Visual Studio 2017 173```batch 174mkdir projects\build 175cd projects\build 176cmake -G "Visual Studio 15" ..\CMake\ 177msbuild Project.sln 178``` 179 180### makefile builds 181 182#### OSX 183```shell 184mkdir projects/build 185cd projects/build 186cmake -G"Unix Makefiles" -DCMAKE_BUILD_TYPE=RelWithDebInfo -B. ../CMake 187make 188``` 189 190#### Linux --- Clang 191```shell 192mkdir projects/build 193cd projects/build 194cmake -G"Unix Makefiles" -DCMAKE_C_COMPILER=clang -DCMAKE_CXX_COMPILER=clang++ -DCMAKE_BUILD_TYPE=RelWithDebInfo -DCMAKE_EXE_LINKER_FLAGS="-stdlib=libc++" -B. ../CMake 195make 196``` 197 198#### Linux --- GCC 199```shell 200mkdir projects/build 201cd projects/build 202cmake -G"Unix Makefiles" -DCMAKE_C_COMPILER=gcc -DCMAKE_CXX_COMPILER=g++ -DCMAKE_BUILD_TYPE=RelWithDebInfo -B. ../CMake 203make 204``` 205 206#### Windows 207```batch 208mkdir projects\build 209cd projects\build 210cmake -G"NMake Makefiles" -DCMAKE_BUILD_TYPE=RelWithDebInfo -B. ..\CMake 211nmake 212``` 213 214The build only produces test and example binaries. 215 216# Running tests 217 218* You can use the CMake test runner `ctest` 219* You can run the test binaries directly `rxcpp_test_*` 220* Tests can be selected by name or tag 221Example of by-tag 222 223`rxcpp_test_subscription [perf]` 224 225# Documentation 226 227RxCpp uses Doxygen to generate project [documentation](http://reactivex.github.io/RxCpp). 228 229When Doxygen+Graphviz is installed, CMake creates a special build task named `doc`. It creates actual documentation and puts it to `projects/doxygen/html/` folder, which can be published to the `gh-pages` branch. Each merged pull request will build the docs and publish them. 230 231[Developers Material](DeveloperManual.md) 232 233# Contributing Code 234 235Before submitting a feature or substantial code contribution please discuss it with the team and ensure it follows the product roadmap. Note that all code submissions will be rigorously reviewed and tested by the Rx Team, and only those that meet an extremely high bar for both quality and design/roadmap appropriateness will be merged into the source. 236 237# Microsoft Open Source Code of Conduct 238This project has adopted the [Microsoft Open Source Code of Conduct](https://opensource.microsoft.com/codeofconduct/). For more information see the [Code of Conduct FAQ](https://opensource.microsoft.com/codeofconduct/faq/) or contact [opencode@microsoft.com](mailto:opencode@microsoft.com) with any additional questions or comments. 239